I have an alternative approach to this question.
I started by asking ChatGPT to write me the code.
It didn't quite get it to work and I gave up on reprompting it.
This is one of the versions of the code it wrote:
If you want to manually enter the page number for pagination, you can modify the script to accept user input. Here's how you can do it:
```python
import os
import requests
import csv
# Replace these variables with your Canvas API token and base URL
API_TOKEN = 'your_api_token_here'
BASE_URL = 'https://your_canvas_instance_url/api/v1/'
# Set up headers with Authorization token
headers = {
'Authorization': 'Bearer ' + API_TOKEN,
}
# Manually enter the page number for pagination
page_number = input("Enter the page number: ")
# API endpoint to list courses with per_page=100
url = f"{BASE_URL}courses?per_page=100&page={page_number}"
# Make a GET request to the Canvas API
response = requests.get(url, headers=headers)
# Check if the request was successful (status code 200)
if response.status_code == 200:
# Parse JSON response
courses = response.json()
# Determine the next CSV file number
csv_number = 1
while os.path.exists(f"courses_{csv_number:03d}.csv"):
csv_number += 1
# Write course data to CSV file
csv_filename = f"courses_{csv_number:03d}.csv"
with open(csv_filename, 'w', newline='', encoding='utf-8') as csvfile:
csv_writer = csv.writer(csvfile)
csv_writer.writerow(['Course Number', 'Course Name'])
# Iterate over courses and write to CSV
for course in courses:
csv_writer.writerow([course['id'], course['name']])
print(f"Course data saved to {csv_filename}")
else:
print(f"Failed to retrieve courses. Status code: {response.status_code}")
```
This script will prompt you to manually enter the page number for pagination. Based on the provided page number, it will fetch the courses from the Canvas API and save them to a CSV file with the appropriate course number and name columns.
I then asked Canvas support for help with the API and they came up with this:
Agent Brett H
( 1s ) Brett H: Hi Rupert! How can I help you today?
( 52s ) Rupert: Hi I am trying to get a list of all the canvas units at ACU. I am wanting the canvas unit number & name
( 1m 18s ) Brett H: Ok, when you say unit are you referring to a canvas course ?
( 1m 18s ) Rupert: I am playing with API calls which is partly working but it stops at 32 courses
( 1m 33s ) Rupert: Sorry yes Course
( 1m 53s ) Rupert: (our terminology is unit)
( 2m 58s ) Brett H: Ok, So I do not have an API call but you can certainly run a report here in your instance that will pull that information:https://canvas.acu.edu.au/accounts/self/settings#tab-reports
( 3m 39s ) Brett H: I would recommend a 'Provisioning report' and configure that to courses
Thanks!
Canvas Support

I then went to ask canvas support for help on the API pagination issue and started off with the broad question of how to get a list of all the Canvas Courses. This is what they came up with and it works!
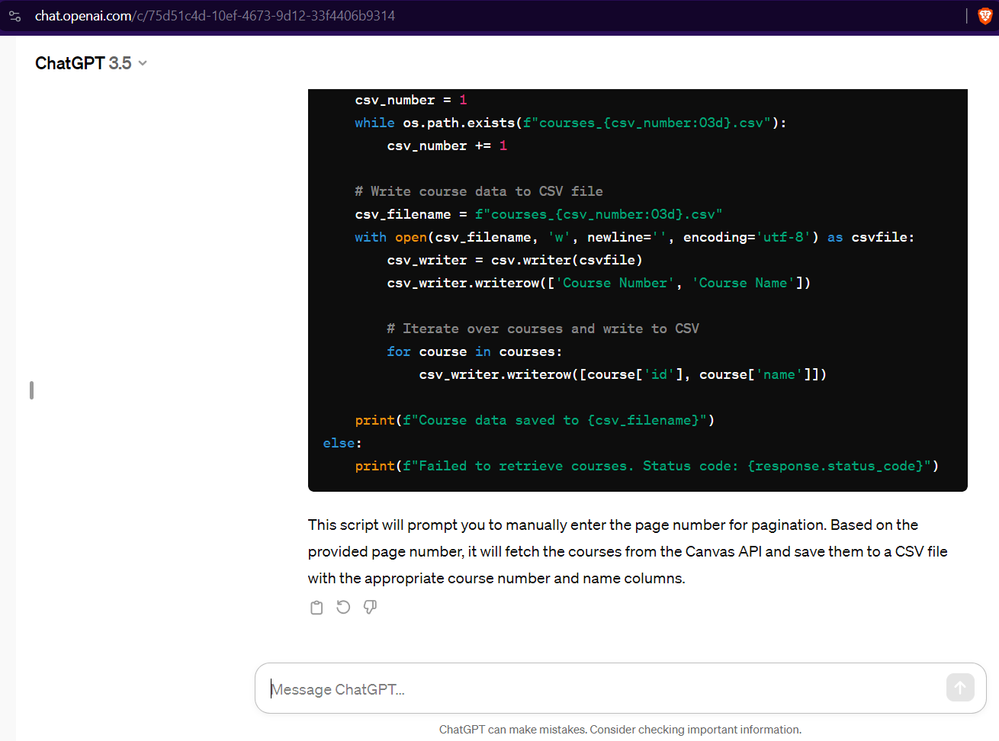