We decided to developed a quick online tool to help us troubleshoot user issues.
We will be getting our learners to run this tool and report the results to us if they encounter problems accessing Canvas.
In this way, we will have sufficient info to better advise them on the next course of actions.
On Desktop
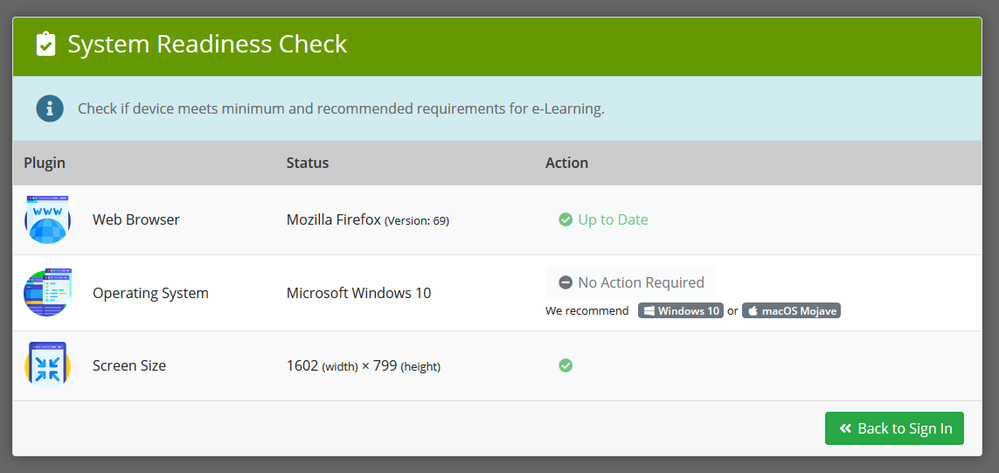
On Mobile:

We make use of JQuery Device Detector to develop this tool. We evaluated numerous JQuery solutions and decided on this plugin: JS Device Detector.
The HTML of the tool is as follows:
<div class="container h-100">
<div class="row h-100 justify-content-center align-items-center">
<div class="col-12">
<div class="card">
<div class="card-header"><h3><em class="fas fa-fw fa-clipboard-check"></em> System Readiness Check</h3></div>
<div class="card-body card-alert">
<div class="alert alert-info"><em class="fa fa-info-circle fa-2x"></em> <span>Check if device meets minimum and recommended requirements for e-Learning.</span></div>
</div>
<div class="table-responsive-sm">
<table class="card-table table table-striped table-hover">
<thead class="thead-dark">
<tr>
<th scope="col" style="width: 55px;">Plugin</th>
<th scope="col"></th> <th scope="col">Status</th>
<th scope="col">Action</th>
</tr>
</thead>
<tbody>
<tr class="browserRow">
<td class="align-middle"><img alt="Web Browser" src="/img/browser.png" width="55" /></td>
<td class="align-middle">Web Browser</td> <td class="align-middle"><span></span> <small>(Version: <span></span>)</small></td>
<td class="align-middle"><span></span></td>
</tr>
<tr>
<td class="align-middle"><img alt="Operating System" src="/img/os.png" width="55" /></td>
<td class="align-middle">Operating System</td> <td class="align-middle"><span></span></td>
<td class="align-middle"><span></span> <a class="btn btn-light disabled" href="#"><em class="fas fa-fw fa-minus-circle"></em> No Action Required</a> <br /> <small>We recommend </small> <a class="badge badge-secondary" href="https://www.microsoft.com/en-sg/p/windows-10-home/d76qx4bznwk4?icid=All_WindowsCat_0518&activetab=pivot%3aoverviewtab" target="_blank"><em class="fab fa-fw fa-windows"></em> Windows 10</a> <small>or</small> <a class="badge badge-secondary" href="https://apps.apple.com/sg/app/macos-mojave/id1398502828?mt=12&ign-mpt=uo%3D4" target="_blank"><em class="fab fa-fw fa-apple"></em> macOS Mojave</a></td>
</tr>
<tr>
<td class="align-middle"><img alt="Screen Size" src="/img/screensize.png" width="55" /></td>
<td class="align-middle">Screen Size</td>
<td class="align-middle"><span></span></td> <td class="align-middle"><span></span></td>
</tr>
</tbody>
</table>
</div>
<div class="card-footer"><a class="btn btn-success float-right" href="https://lms.learnforlife.sg"><em class="fas fa-fw fa-angle-double-left"></em> Back to Sign In</a></div>
</div>
</div>
</div>
</div>
The script to initialise the plugin is as follows:
// Check Browser, OS and Devices
$(document).ready(updateDomContent);
$(window).on('resize', updateDomContent);
function updateDomContent() {
var d = $.fn.deviceDetector; // eslint-disable-line no-var
var userAgentString = navigator.userAgent || // eslint-disable-line no-var
navigator.appVersion;
$('#useragent').text(userAgentString);
// Info
$('#info').text(JSON.stringify(d.getInfo(), false, 4));
// General
$('#supported').text(d.isSupported());
$('#mobile').text(d.isMobile());
$('#desktop').text(d.isDesktop());
// Browser
$('#browserVersion').text(d.getBrowserVersion());
$('#browserName').text(d.getBrowserName());
$('#browserId').text(d.getBrowserId());
// OS
$('#osVersion').text(d.getOsVersion());
$('#osVersionString').text(d.getOsVersionString());
$('#osVersionCategories').text(JSON.stringify(d.getOsVersionCategories()));
$('#osVersionMajor').text(d.getOsVersionMajor());
$('#osVersionMinor').text(d.getOsVersionMinor());
$('#osVersionBugfix').text(d.getOsVersionBugfix());
$('#osName').text(d.getOsName());
$('#osId').text(d.getOsId());
// Apple
$('#safari').text(d.isSafari());
$('#iphone').text(d.isIphone());
$('#ipad').text(d.isIpad());
$('#ios').text(d.isIos());
$('#macos').text(d.isMacos());
// Google
$('#chrome').text(d.isChrome());
$('#android').text(d.isAndroid());
// Mozilla
$('#firefox').text(d.isFirefox());
// Microsoft
$('#ie').text(d.isIe());
$('#msie').text(d.isMsie());
$('#edge').text(d.isEdge());
$('#ieMobile').text(d.isIeMobile());
$('#windowsPhone').text(d.isWindowsPhone());
$('#windows').text(d.isWindows());
// Opera
$('#opera').text(d.isOpera());
$('#operaMini').text(d.isOperaMini());
// BlackBerry
$('#blackberry').text(d.isBlackberry);
// other
$('#linux').text(d.isLinux());
$('#bsd').text(d.isBsd());
// Write Info
$('span').each(function() {
var _this = $(this); // eslint-disable-line no-var, no-invalid-this
var status = _this.text(); // eslint-disable-line no-var
_this.attr('data-status', status);
});
// Browser Actions
if (d.getBrowserId() == 'firefox' && d.getBrowserVersion() < 67) {
$('#browserAction').html('<a role="button" class="btn btn-warning" href="https://www.mozilla.org/en-US/firefox/new/" target="_blank"><i class="fas fa-fw fa-exclamation-circle"></i> Update Browser</a>');
} else if (d.getBrowserId() == 'chrome' && d.getBrowserVersion() < 75) {
$('#browserAction').html('<a role="button" class="btn btn-warning" href="https://www.google.com/chrome/" target="_blank"><i class="fas fa-fw fa-exclamation-circle"></i> Update Browser</a>');
} else if (d.getBrowserId() == 'safari' && d.getBrowserVersion() < 11) {
$('#browserAction').html('<a role="button" class="btn btn-warning" href="https://www.apple.com/sg/macos/how-to-upgrade/" target="_blank"><i class="fas fa-fw fa-exclamation-circle"></i> Update Mac OS</a>');
} else if (d.getBrowserId() == 'edge' && d.getBrowserVersion() < 44) {
$('#browserAction').html('<a role="button" class="btn btn-warning" href="https://www.whatismybrowser.com/guides/how-to-update-your-browser/edge" target="_blank"><i class="fas fa-fw fa-exclamation-circle"></i> Update Windows OS</a>');
} else if (d.getBrowserId() == 'msie' && d.getBrowserVersion() <= 11) {
$('#browserAction').html('<a role="button" class="btn btn-light disabled text-danger" href="#"><i class="fas fa-fw fa-exclamation-circle"></i> You are using an old web browser.</a><br><small>Use these web browsers instead:</small> <a class="badge badge-secondary" href="https://www.mozilla.org/en-US/firefox/new/" target="_blank"><i class="fab fa-fw fa-firefox"></i> Firefox</a> <a class="badge badge-secondary" href="https://www.google.com/chrome/" target="_blank"><i class="fab fa-fw fa-chrome"></i> Chrome</a>');
} else {
$('#browserAction').html('<a role="button" class="btn btn-light disabled text-success" href="#"><i class="fas fa-fw fa-check-circle"></i> Up to Date</a>');
}
}
// Check Screen Size
var WindowsSize=function(){
var h=$(window).height(),
w=$(window).width();
$("#winSize").html(+w+" <small>(width)</small> × "+h+" <small>(height)</small>");
if ( $(window).width() > 1023 ) {
$('#screenAction').html('<a role="button" class="btn btn-light disabled text-success" href="#"><i class="fas fa-fw fa-check-circle"></i></a>');
}
else {
$('#screenAction').html('<a role="button" class="btn btn-light disabled text-danger" href="#"><i class="fas fa-fw fa-exclamation-circle"></i> We recommend using <strong>tablet or larger device</strong> in <strong>landscape orientation</strong> for e-Learning</a>');
}
};
$(document).ready(WindowsSize);
$(window).resize(WindowsSize);
The tool is dependent on Jquery, Bootstrap 4 and Fontawesome 5, which can be loaded from CDNs.
Hope this will be useful to those who need it. 
This discussion post is outdated and has been archived. Please use the Community question forums and official documentation for the most current and accurate information.